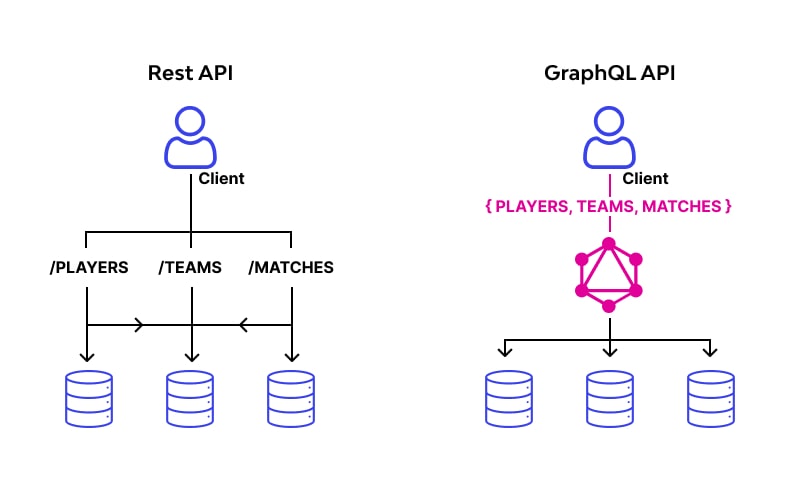
=> Overview :-
• An API (Application Programming Interface) allows different software applications to communicate with each other.
It specifies how software components should interact, and there are two common types of APIs in web development: REST and GraphQL.
=> REST API Overview :-
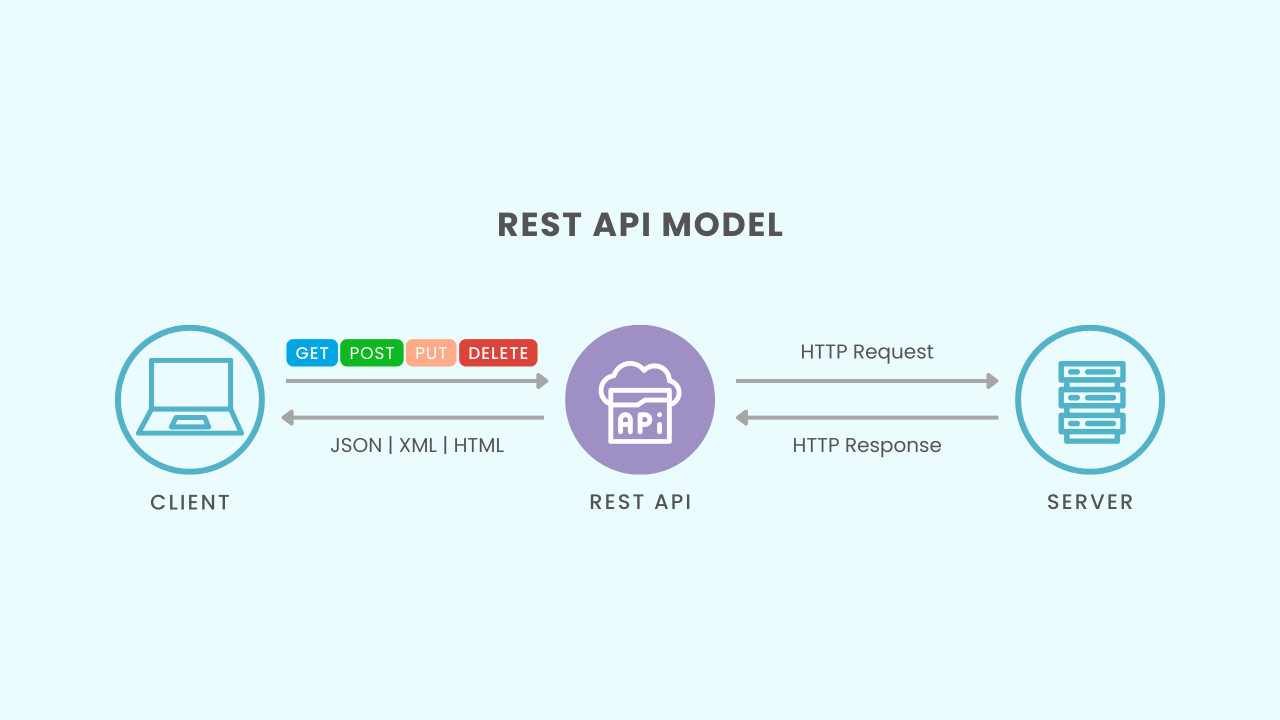
• REST (Representational State Transfer) is an architectural style for designing networked applications.
It operates over HTTP and is based on stateless interactions, meaning that each API call is
independent, and the server doesn’t store any client state between requests.
=> Key Characteristics of REST :-
-> Resources:
• Represented by URLs (e.g., https://api.example.com/users).
-> HTTP Methods :-
• GET: Retrieve Data
– Used to fetch data from the server.
– Does not modify the resource, making it an idempotent operation (calling it multiple times produces the same result).
• POST: Create New Data
– Used to create a new resource on the server.
– Sends data in the request body.
• PUT/PATCH: Update Existing Data
– PUT: Replaces the entire resource.
– PATCH: Updates only specified fields in the resource.
• DELETE: Remove Data
– Used to delete a resource from the server.
-> Stateless:-
• Statelessness means that each request from the client to the server must contain all the
necessary information to understand and process the request.
-> Benefits:
• Scalability: No dependency on server memory.
• Simplicity: No need for session management.
-> Client-Server Architecture:
• REST is based on a client-server model, where the client and server have distinct roles:
– Client: Responsible for the user interface and user experience.
– Server: Responsible for handling requests and managing resources.
• Separation of concerns:
– Clients do not need to know how data is stored or processed on the server.
– Servers do not need to know how the data is presented to the end-user.
=> GraphQL Overview:-
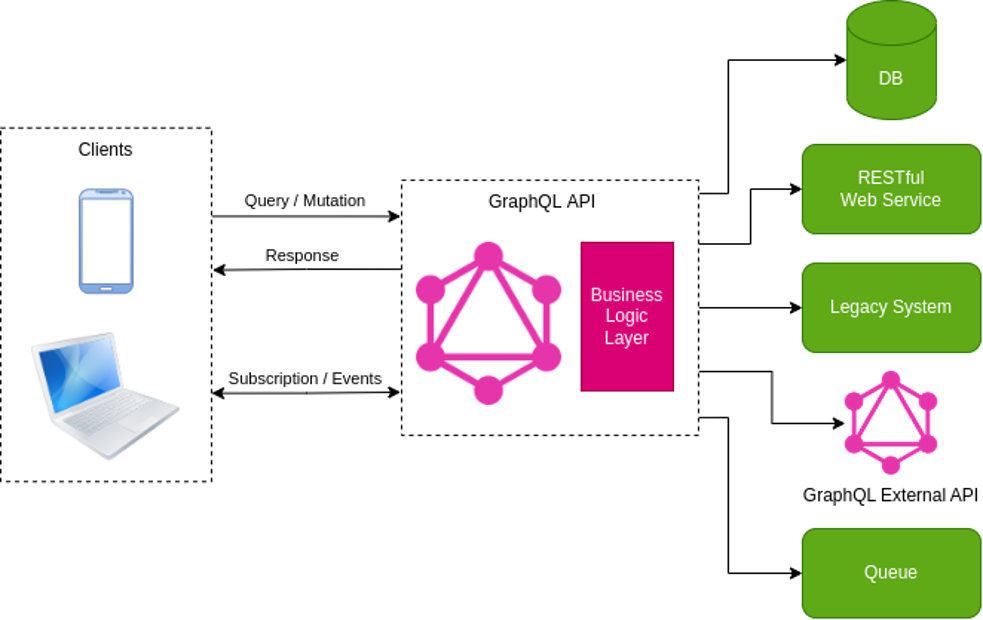
• GraphQL is a query language for APIs and a runtime for fulfilling those queries with your existing data.
GraphQL provides a complete and understandable description of the data in your API, gives clients the power to
ask for exactly what they need and nothing more, makes it easier to evolve APIs over time, and enables powerful developer tools.
=> Key Characteristics of GraphQL:-
-> Single Endpoint:
• Unlike REST, which typically has multiple endpoints (e.g., /users, /posts),
GraphQL uses a single endpoint for all queries, mutations, and subscriptions.
• The client sends a request to the GraphQL server, specifying what data it needs or what actions it wants to perform.
-> Queries:
• GraphQL queries allow clients to specify exactly the data they need.
The server responds with only the requested fields, avoiding over-fetching or under-fetching data.
• Clients can request nested or related data in a single query.
-> Mutations
• Mutations are used to modify or update data on the server, such as creating, updating, or deleting resources.
• Mutations can also return data, allowing clients to know the result of their action immediately.
-> Subscriptions
• Subscriptions enable real-time communication between the server and clients.
They are used to receive updates when specific events occur (e.g., new messages, status changes).
• Typically implemented using WebSockets.
-> Strongly Typed Schema
• A GraphQL schema defines the structure of the data, including the types, queries, mutations, and subscriptions.
• It acts as a contract between the client and server, ensuring both sides understand the data model and operations.
= > Key Differences Between REST and GraphQL
-> REST API
• Architecture:- Multiple endpoints (e.g., /users)
• Data Fetching:- Fixed responses, over-fetching/under-fetching possible
• Performance:- Multiple requests required for different resources
• Versioning:- New versions of API need to be created (e.g., v1, v2)
• Query Complexity:- Limited flexibility for complex queries
• Response Format:- Usually JSON
-> GraphQL
• Architecture:- Single endpoint (/graphql)
• Data Fetching:- Clients control exactly what data they need
• Performance:- Single request fetches all needed data
• Versioning:- No versioning needed, schema evolution handled dynamically
• Query Complexity:- Allows complex, nested queries
• Response Format:- JSON with a flexible structure
=> When to Use REST vs GraphQL? :-
-> REST :-
• Simple CRUD operations.
• When your application doesn’t require flexibility in data fetching.
• When the data model is simple and doesn’t change frequently.
-> GraphQL :-
• Complex and dynamic data structures.
• When clients need fine-grained control over the data they request.
• When reducing the number of requests is important (e.g., mobile apps).
=> Advanced Concepts:-
• GraphQL Subscriptions: Real-time updates sent from the server to the client.
• REST Pagination: Typically implemented using query parameters like page and limit.
• Rate Limiting in REST: Protects API endpoints from being overwhelmed by too many requests.
• Error Handling: REST usually uses HTTP status codes for errors, while GraphQL can return custom error messages in the response body.
=> Conclusion:-
• Both REST and GraphQL are powerful tools for building APIs, but they have different strengths. REST is simple and straightforward,
while GraphQL provides more flexibility, particularly when dealing with complex data and when performance optimization is a priority.
Choose based on your application’s needs.